Overview
BookSquirrel(v1.4) is for those who want to keep a record of books they’ve read. More importantly, BookSquirrel is optimized for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI). If you can type fast, BookSquirrel can get your note management tasks done faster than traditional GUI apps.
Summary of contributions
-
Major enhancement: added the Review feature.
-
What it does: allows the user to add, list, view, and delete reviews.
-
Justification: This feature enables the user to record their thoughts when they read books.
-
Highlights: This feature requires a lot of change to the UI and model of the application, meanwhile, it is closely linked to the book feature, which makes it challenging and bug-prone. To find a way to store reviews, many architectures were considered. Extra care was taken to integrate Reviews with existing Book commands, such as to cascade any deletion or editing of books.
-
-
Minor enhancement: fixed the bug that ConfigUtil will always output config.json with missing new line at end of file, which causes the travis build to fail unless a new line is manually added.
-
Code contributed: [Functional code]
-
Other contributions:
-
Project management:
-
Created and managed the Project board
-
Created and organized tags
-
-
Enhancements to existing features:
-
Wrote additional tests for existing features to increase coverage from 87% to 92% (Pull request #95)
-
-
Documentation:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Review Commands
Adding a book review: addReview
Adds a book review to a certain book in the Bookshelf
Format: addReview n/BOOKNAME rt/REVIEW TITLE r/REVIEW MESSAGE
Examples:
-
addReview n/Alice in Wonderland rt/An interesting child book r/While Lewis Carroll purists will scoff at the aging of his curious young protagonist, most movie audiences will enjoy this colorful world.
-
addReview n/Structure and Interpretation of Computer Programs rt/Computing Bible r/A very difficult book:(
Deleting a book review: deleteReview
Deletes the review of the specified index number from the review list.
Format: deleteReview INDEX
Example:
-
deleteReview 1
Listing all book reviews: listAllReviews
Lists all the reviews in the BookShelf.
Format: listAllReviews
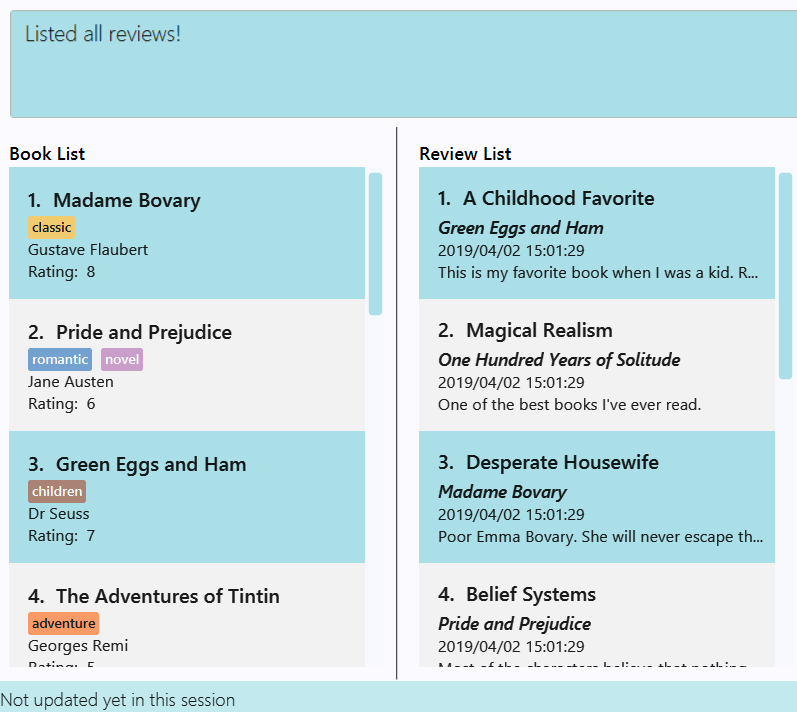
Listing book reviews of a certain book : listReview
Lists the reviews of the book identified by the index number used in the displayed Bookshelf.
Format: listReview INDEX
Example:
-
listReview 1
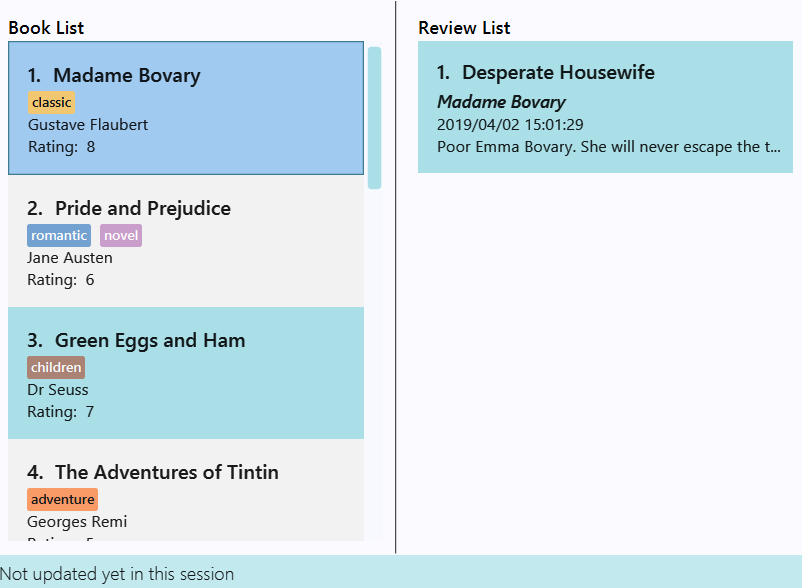
Selecting an review: selectReview
Selects a review.
Format: selectReview INDEX
Example:
-
selectReview 1
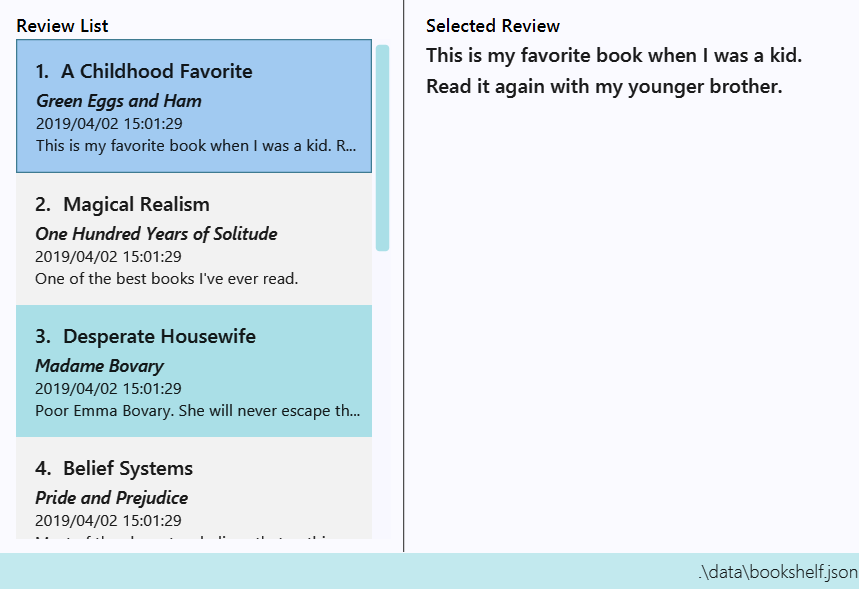
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
UI component
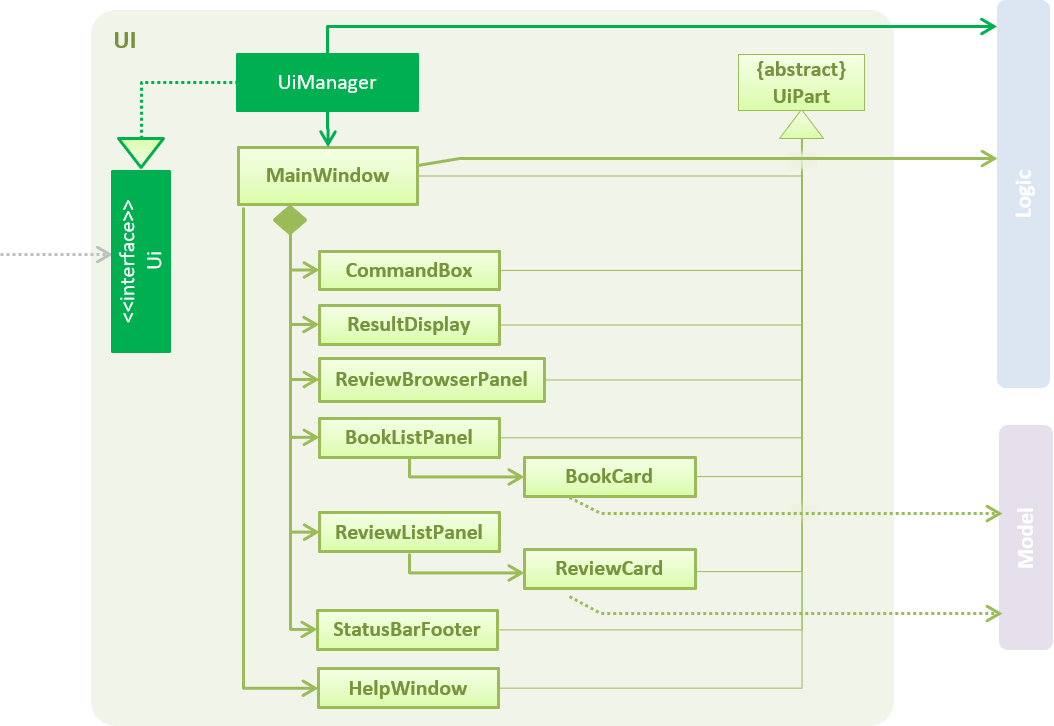
API : Ui.java
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("deleteBook 1")
API call.
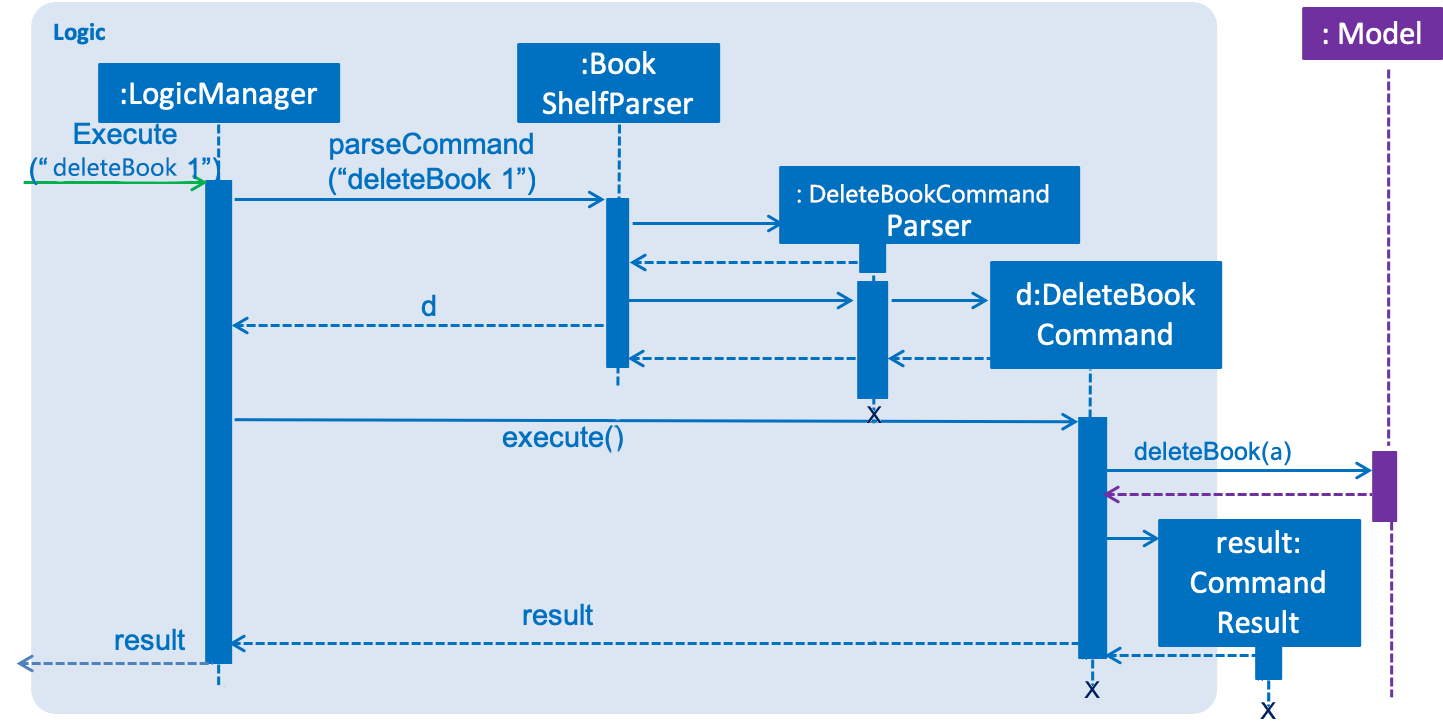
deleteBook 1
CommandAddReview feature
Current Implementation
Given below is an example usage and how addReview feature behaves at each step.
Step 1. The user launches the application for the first time. The VersionedBookShelf
will be initialized with the initial bookshelf state, and the currentStatePointer
pointing to that single bookshelf state.
Step 2. The user executes addReview n/Alice in Wonderland rt/Carroll has depicted a unique world I hadn’t seen before r/Alice’s Adventures in Wonderland by Lewis Carroll is a story about Alice who falls down a rabbit hole and lands into a fantasy world that is full of weird, wonderful people and animals.
Step 3. The AddReviewCommandParser
parses this command and creates a Review
object based on the parameters in user input.
Step 4. The AddReviewCommandParser`returns an `AddReviewCommand
. The exception will be thrown if the command is invalid.
Step 5. The AddReviewCommand
executes. The command checks if the book to which the review is added exists in the BookShelf
, based on BookNameContainsExactKeywordPredicate
. If the book does not exist, an exception is thrown.
Step 6. If the book exists, the review is added to the review list of the Bookshelf.
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command addBook n/Alice rt/rt/interesting r/an interesting story book…

Design Considerations
Aspect: Data structure to store Reviews
-
Alternative 1 (current choice): Use a List to store Reviews
-
Pros: There will be an order for the reviews added based on time created. More clear to users.
-
Cons: May have unforeseen bugs or implications.
-
-
Alternative 2: Use a Set to store all Reviews, just like tags.
-
Pros: Easy to code and manage because it is the same implementation as tags.
-
Cons: Set does not allow duplicate reviews. Need to check for duplicate reviews added.
-
Aspect: Where to store Reviews
-
Alternative 1 (current choice): Have another independent list for reviews in the bookshelf.
-
Pros: Much easier to implement.
-
Cons: Reviews and Books now have exactly the same structure, which violates the DRY principle.
-
-
Alternative 2: Use a Set within a Book, just like tags.
-
Pros: The model makes more sense because Reviews belong to Books.
-
Cons: Makes it more difficult to link with the UI component.
-
Aspect: Constraints on Review Parameters
-
ReviewTitle has the same constraints as BookName, less than 50 characters.
-
ReviewMessage should be less than or equal to 400 characters. This is because of the BookBrowserPanel used to display the ReviewMessage currently cannot hold more than 400 characters. The longer text also makes it more difficult to edit in the small CommandBox. Future releases would consider adding enhanced review input panel to make the review feature more user-friendly.
Aspect: Auto-creation of Date for the Review
-
Currently, the constructor of Review automatically assigns a dateCreated to it. This feature is provided for the convenience of the user. An overloaded constructor is available where the date can be passed in as a parameter, to be used in testing.
ListReview feature
Current Implementation
Given below are an example of usage and program behavior:
Step 1. The user executes listReview 1
Step 2. The ListReviewCommandParser parses this command and returns a new ListReviewCommand with the specified index.
Step 3. The ListReviewCommand executes. The book with the specified index is selected.
Step 4. Reviews of the selected book will be displayed in the rightmost panel.
Alternatively, the user can click the book to select it.
ListAllReviews feature
Current Implementation
The ListAllReviews Feature uses the same implementation as the ListBook feature:
Step 1. The user executes listAllReviews
Step 2. A new ListAllReviewsCommand is returned by the BookShelfParser
Step 3. The ListAllReviewsCommand executes. Review list panel is updated to show all reviews.
Design Considerations
Aspect: Whether to combine ListAllReviews command with ListReview command
-
Alternative 1 (current choice): not to combine.
-
Pros: Command is more logical and user-friendly because it is actually not intuitive to have two functions combined in one ListReview command.
-
Cons: Inconsistency with the ListBook command may cause confusion.
-
-
Alternative 2: Combine.
-
Pros: Consistency.
-
Cons: Command is not intuitive, and takes extra time to implement review predicates.
-
SelectReview feature
Current Implementation
Selection of review is implemented the same way as the selection of a book.
In addition, review message of a selected review will be displayed in the book browser panel.
Step 1. The user executes selectReview 1
Step 2. The SelectReviewCommandParser parses this command and returns a new SelectReviewCommand with index 1.
Step 3. The SelectReviewCommand executes. The review with the specified index is selected.
Step 4. The BookBrowserPanel listens to the change in the selected review and displays its review message.
DeleteReview feature
Current Implementation
The review is deleted based on the index.
Step 1. The user executes deleteReview 1
Step 2. The DeleteReviewCommandParser parses this command and returns a new DeleteReviewCommand with index 1.
Step 3. The DeleteReviewCommand executes. The review with the specified index is deleted.
Other Design Considerations
Whether to Implement EditReview
It seems natural to implement EditReview as one component of the CRUD features. However, editing an existing review requires re-typing everything and seems painstaking to the user. It makes much more sense to just delete the original review and add a new one. Hence. EditReview is currently not implemented.
Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
Adding a review
-
Adding a review with the target book existing in bookshelf
-
Test case:
addReview n/Pride and Prejudice rt/Belief Systems r/Most of the characters believe that nothing matters more than social class…
Expected: a review titled "Belief Systems" should appear in the review list.
-
-
Adding a review with the target book not existing in bookshelf
-
Test case:
addReview n/Invalid Bookname rt/Belief Systems r/Most of the characters believe that nothing matters more than social class…
Expected: the following error message should appear: "The target book does not exist in the book shelf".
-
-
Other invalid commands to try:
-
Test case:
addReview n/Pride and Prejudice rt/Belief%Systems r/Most of the characters believe that nothing matters more than social class…
Expected: error message: "Review titles should contains only alphanumeric characters, spaces, '*', ',', '.', '?', ''', '()' and '&'. And it should not be blank or have more than 50 characters (space included).". -
Test case:
addReview rt/Belief Systems r/Most of the characters believe that nothing matters more than social class…
Expected: error message: "Invalid command format! addReview: Adds a review to a certain book. Parameters: n/BOOK NAME rt/REVIEW TITLE r/REVIEW Example: addReview n/Alice in Wonderland rt/A great fairytale r/While Lewis Carroll purists will scoff at the aging of his young protagonist…" -
Test case:
addReview n/Pride and Prejudice rt/Belief Systemssssssssssssssssssssssssssssssssssssss r/Most of the characters believe that nothing matters more than social class…
Expected: error message: "Review titles should contains only alphanumeric characters, spaces, '*', ',', '.', '?', ''', '()' and '&'. And it should not be blank or have more than 50 characters (space included).".
-
Deleting a review
-
Deleting a review while all reviews are listed
-
Prerequisites: List all reviews using the
listAllReviews
command. All reviews present in the bookshelf are shown in the list. -
Test case:
deleteReview 1
Expected: First review is deleted from the list. Timestamp in the status bar is updated. -
Test case:
deleteReview 0
Expected: No review is deleted. Error details are shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
deleteReview
Expected: Similar to previous.
-
Listing reviews
-
Listing a review of a book
-
Prerequisites: 1 or more books are listed in the book list, by the listBook or sortBook command.
-
Test case:
listReview 1
Expected: the book with the index is selected. Reviews to that book are listed in the review list. -
Test case:
listReview 0
Expected: Error details are shown in the status message. Status bar remains the same.
-
-
Listing all reviews
-
Test case:
listAllReviews
Expected: any selected book is deselected. All reviews in the book shelf are listed in the review list.
-
Selecting review
-
Selecting a review while all reviews are listed
-
Prerequisites: List all reviews using the
listAllReviews
command. All reviews present in the bookshelf are shown in the list. -
Test case:
selectReview 1
Expected: First review is selected. The complete review is shown in the selected review panel. -
Test case:
selectReview 0
Expected: No review is selected. Error details are shown in the status message. -
Other incorrect delete commands to try:
selectReview
Expected: Similar to previous.
-